Developers want to move fast. 🚀 🚀 🚀 Manually triggering a rebuild of your source code after ever little change is slow and annoying. With the WebpackDev Server and Webpack watch we can greatly improve our code, build, try cycles. We'll use the existing code from the Getting Started with Webpack: TypeScript blog post as a starting point.
- Provide an abstraction for webpack with the ability for other modules to extend the webpack config, with integration into the flamingo-carotene-dev-server module.
- Webpack webpack-dev-server problem matcher for vscode - tasks.json.

Under the hood, Webpack dev server is a mini Node.js Express server. It uses a library called SockJS to emulate a web socket. The Node.js server listens to when files were changed, and triggers events to react accordingly. Webpack dev server is also a separate package that needs to get install via NPM.
For this guide we'll be using Node v8.5, NPM v5.5, TypeScript v2.5, and Webpack v3.8. The following commands will tell you which versions you have installed. If they are not exact they may still work. TypeScript and Webpack will be installed a little further on.
You might notice that Webpack is at a different version than the previous blog post. They are constantly adding features and fixing bugs so they release pretty often. The easy way to update your dependancies is with npm update
from within the application directory.
Currently we have the command npm run build
to compile our code and npm run serve
to run a development server on http://localhost:8080
. Every time you make a change to the source code you have to manually execute npm run build
. Let's simplify this so that you just have npm run serve
and build will automatically run when code changes.
The first step is to get builds happening automatically when code changes. With Webpack that's quite easy to accomplish with the --watch
flag. Update the package.json
to have a new watch
script.
Now if you run npm run watch
you'll notice that the command never ends. That's because Webpack is 'watching' your source code for changes.
Why not update build
to include --watch
? Sometimes you want to run a one-off build and if you combine them you have to start the watch, let it build once, and then close it.
If you make a change to index.ts
and save the file you'll see the file get built again.
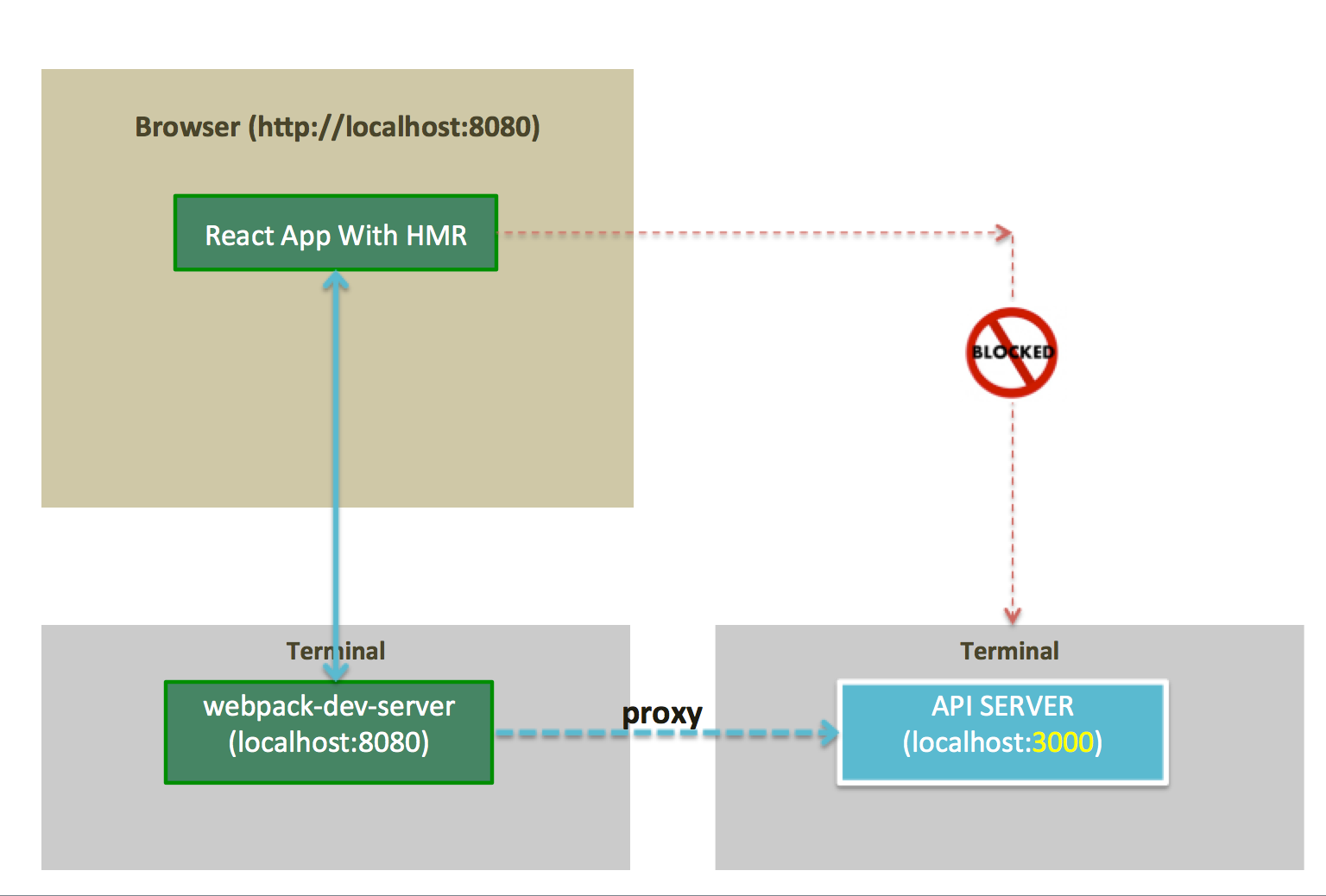
To get an initial combined command is actually very easy. Change the serve
script command to look like the following. The single &
will tell most systems to run the command before and after it in parallel.
You can run a single command npm run serve
and Webpack will be watching for and recompiling code changes in one thread and http-server
will be serving those results in another thread. One downside to this is that both TypeScript compilation messages and http-server messages will mix with each other in the output.
You could leave the setup like this but moving to the Webpack Dev Server will enable some pretty hot features we'll cover in future blog posts so let's go ahead and do that now.
Add the webpack-dev-server
package.
You'll need to tell the Dev Server what to serve. Currently we are serving the index.html
file from the root of the directory.
Next update the package.json
serve
command again, this time to use the new Dev Server.
Note the --open
flag, this will tell Webpack to open the site in a browser window.
It's so much work 👩💻 though to have to still reload the site in the browser every time you make a change. Let's kick it up a notch and add some Hot Module Replacement #magic 🕴. You'll need the html-webpack-plugin
module installed first.
To enable HMR make a couple of changes to webpack.config.js
.
Require the needed packages:
Enable hot
for the devServer
:
Add three plugins:
HotModuleReplacementPlugin
just says 'hey, use the new hotness' and NamedModulesPlugin
cleans up the build logs to only show the entry points we define.
HtmlWebpackPlugin
is a little more interesting. It will take the template
file that is scoped to the root of the directory and output it to the filename
within the dist
directory. The main reason for this is so that Webpack can automatically add the compiled <script>
tags required for HMR.
The final change is to remove <script defer src='dist/bundle.js'></script>
from the index.html
in the root directory. Since Webpack is now adding that automatically we don't want it included multiple times.
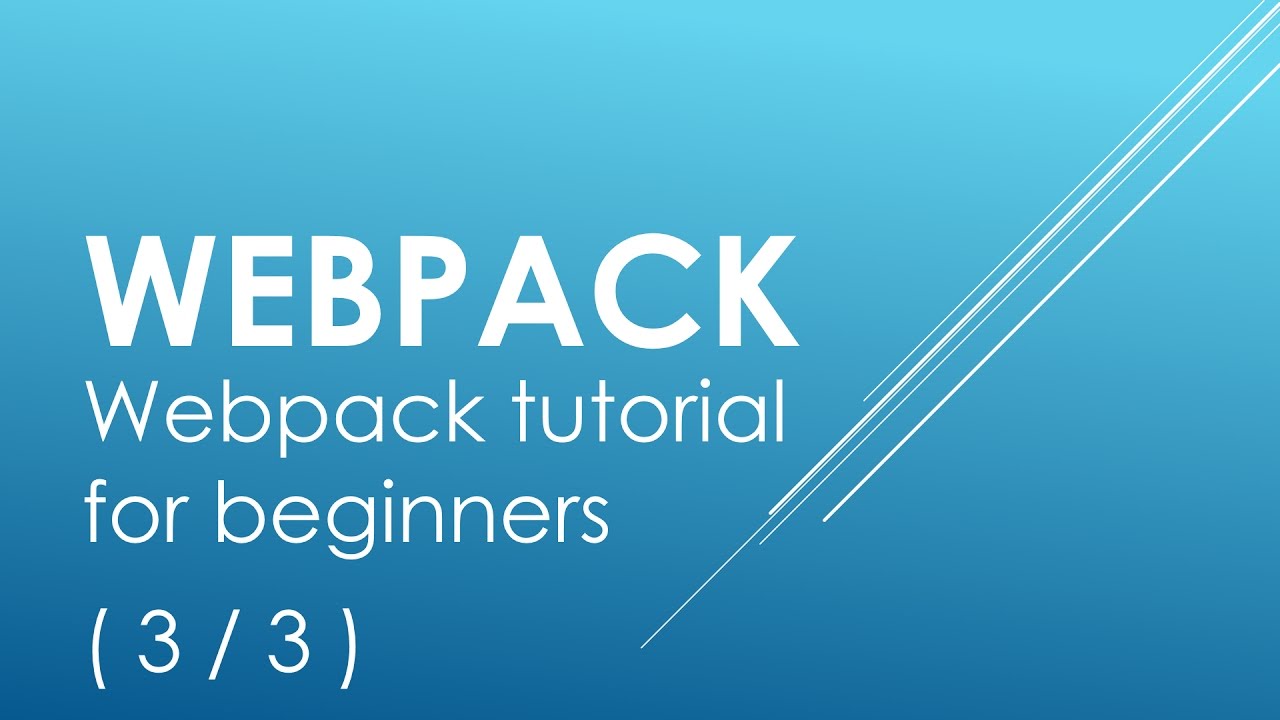
Webpack Dev Server Npm
🏆 Now you can write code, save it, and Webpack will push the changes to the browser without you ever having to leave the editor.
You can view the source for the progress so far on GitHub and check out the next article Getting started with Webpack: Source Maps.
Posts in this series
Use webpack with a development server that provideslive reloading. This should be used for development only.
It uses webpack-dev-middleware under the hood, which providesfast in-memory access to the webpack assets.
Table of Contents
- Usage
Getting Started
First things first, install the module:
Note: While you can install and run webpack-dev-server globally, we recommendinstalling it locally. webpack-dev-server will always use a local installationover a global one.
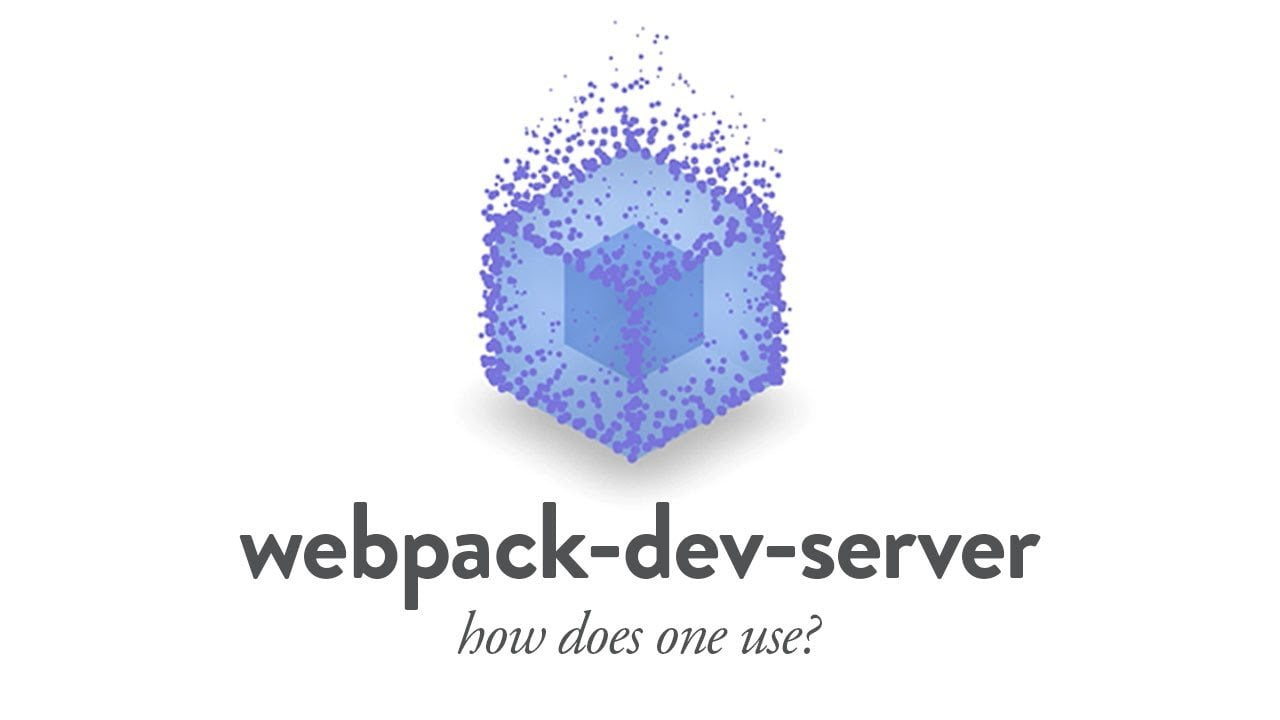
Usage
There are two main, recommended methods of using the module:
With the CLI
The easiest way to use it is with the CLI. In the directory where yourwebpack.config.js
is, run:
Note: Many CLI options are available with webpack-dev-server
. Explore this link.
With NPM Scripts
NPM package.json scripts are a convenient and useful means to run locally installedbinaries without having to be concerned about their full paths. Simply define ascript as such:
And run the following in your terminal/console:
NPM will automagically reference the binary in node_modules
for you, andexecute the file or command.
The Result
Either method will start a server instance and begin listening for connectionsfrom localhost
on port 8080
.
webpack-dev-server is configured by default to support live-reload of files asyou edit your assets while the server is running.
See the documentation for more use cases and options.
Browser Support
While webpack-dev-server
transpiles the client (browser) scripts to an ES5state, the project only officially supports the last two versions of majorbrowsers. We simply don't have the resources to support every whackybrowser out there.
If you find a bug with an obscure / old browser, we would actively welcome aPull Request to resolve the bug.
Webpack Dev Server Port
Support
We do our best to keep Issues in the repository focused on bugs, features, andneeded modifications to the code for the module. Because of that, we ask userswith general support, 'how-to', or 'why isn't this working' questions to try oneof the other support channels that are available.
Your first-stop-shop for support for webpack-dev-server should by the excellentdocumentation for the module. If you see an opportunity for improvementof those docs, please head over to the webpack.js.org repo and open apull request.
From there, we encourage users to visit the webpack Gitter chat andtalk to the fine folks there. If your quest for answers comes up dry in chat,head over to StackOverflow and do a quick search or open a newquestion. Remember; It's always much easier to answer questions that include yourwebpack.config.js
and relevant files!
Webpack Dev Server Webpack 5
If you're twitter-savvy you can tweet #webpack with your questionand someone should be able to reach out and lend a hand.
If you have discovered a
Contributing
Webpack Dev Server Public Path
We welcome your contributions! Please have a read of CONTRIBUTING.md for more information on how to get involved.
Attribution
This project is heavily inspired by peerigon/nof5.